Animated and Responsive Login Form using HTML, CSS and JavaScript
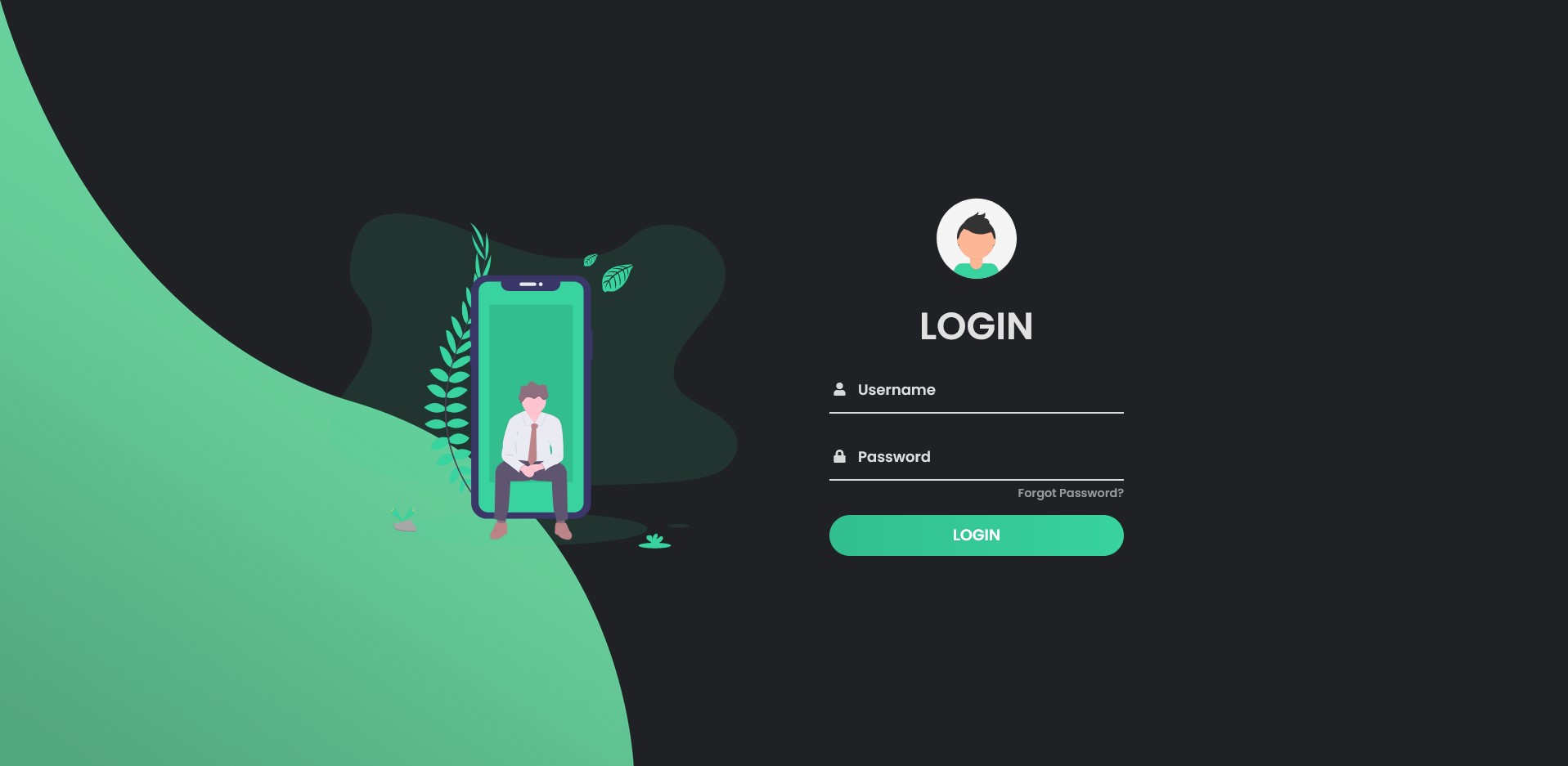
Hello readers, today in this blog I will show you how to create an animated and responsive Login Form only with HTML & CSS. Nearly every website has a login page and that´s why a login form is very useful. It validates the information of an user with various inputs like the email or password input.
In this blog (How to create an Animated and Responsive Login Form using HTML & CSS) we will create a login form which has two inputs. One input for the username and one for the password. Each of them has an icon. There is also a "Forgot Password?" button and a login button. Also there are some design elements next to the form like the profile picture or the phone next to the login form.
If you like this Login Form you can easily get the source code of the project below and use it for your own projects. You can copy the code easily.
Demo
Here is the live demo of "How to create an Animated and Responsive Login Form" if you want to see the final result.
Show Live DemoSource Codes: How to create an Animated and Responsive Login Form
When you want to create this program, first you need to create three files, an HTML file, a CSS file and a JavaScript file. After you have created these files, simply paste the following codes into your file. First, create an HTML file named index.html and paste the given codes into your HTML file. Remember that you must create a file with the .html extension.
<!DOCTYPE html>
<html>
<head>
<title>Animated and Responsive Login Form | CodingWeb</title>
<link rel="stylesheet" type="text/css" href="style.css">
<link href="https://fonts.googleapis.com/css?family=Poppins:600&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/a81368914c.js"></script>
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<img class="wave" src="https://codingweb.blog/demos/Responsive-Login-Form-master/img/wave.png">
<div class="container">
<div class="img">
<img src="https://codingweb.blog/demos/Responsive-Login-Form-master/img/bg.svg">
</div>
<div class="login-content">
<form action="index.html">
<img src="https://codingweb.blog/demos/Responsive-Login-Form-master/img/avatar.svg">
<h2 class="title">Login</h2>
<div class="input-div one">
<div class="i">
<i class="fas fa-user"></i>
</div>
<div class="div">
<h5>Username</h5>
<input type="text" class="input">
</div>
</div>
<div class="input-div pass">
<div class="i">
<i class="fas fa-lock"></i>
</div>
<div class="div">
<h5>Password</h5>
<input type="password" class="input">
</div>
</div>
<a href="#">Forgot Password?</a>
<input type="submit" class="btn" value="Login">
</form>
</div>
</div>
<a href="https://codingweb.blog" target="_blank">Visit CodingWeb for more of this!</a>
<script type="text/javascript" src="main.js"> </script>
</body>
</html>
Next, create a CSS file named style.css
and paste the specified codes into your CSS file. Remember that you must create a file with the extension .css.
*{
padding: 0;
margin: 0;
box-sizing: border-box;
}
body{
font-family: 'Poppins', sans-serif;
overflow: hidden;
background-color: #202124;
}
.wave{
position: fixed;
bottom: 0;
left: 0;
height: 100%;
z-index: -1;
}
.container{
width: 100vw;
height: 100vh;
display: grid;
grid-template-columns: repeat(2, 1fr);
grid-gap :7rem;
padding: 0 2rem;
}
.img{
display: flex;
justify-content: flex-end;
align-items: center;
}
.login-content{
display: flex;
justify-content: flex-start;
align-items: center;
text-align: center;
}
.img img{
width: 500px;
}
form{
width: 360px;
}
.login-content img{
height: 100px;
}
.login-content h2{
margin: 15px 0;
color: #e1e1e1;
text-transform: uppercase;
font-size: 2.9rem;
}
.login-content .input-div{
position: relative;
display: grid;
grid-template-columns: 7% 93%;
margin: 25px 0;
padding: 5px 0;
border-bottom: 2px solid #d9d9d9;
}
.login-content .input-div.one{
margin-top: 0;
}
.i{
color: #d9d9d9;
display: flex;
justify-content: center;
align-items: center;
}
.i i{
transition: .3s;
}
.input-div > div{
position: relative;
height: 45px;
}
.input-div > div > h5{
position: absolute;
left: 10px;
top: 50%;
transform: translateY(-50%);
color: #dcdcdc;
font-size: 18px;
transition: .3s;
}
.input-div:before, .input-div:after{
content: '';
position: absolute;
bottom: -2px;
width: 0%;
height: 2px;
background-color: #38d39f;
transition: .4s;
}
.input-div:before{
right: 50%;
}
.input-div:after{
left: 50%;
}
.input-div.focus:before, .input-div.focus:after{
width: 50%;
}
.input-div.focus > div > h5{
top: -5px;
font-size: 15px;
}
.input-div.focus > .i > i{
color: #38d39f;
}
.input-div > div > input{
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%;
border: none;
outline: none;
background: none;
padding: 0.5rem 0.7rem;
font-size: 1.2rem;
color: #898989;
font-family: 'poppins', sans-serif;
}
.input-div.pass{
margin-bottom: 4px;
}
a{
display: block;
text-align: right;
text-decoration: none;
color: #999;
font-size: 0.9rem;
transition: .3s;
}
a:hover{
color: #38d39f;
}
.btn{
display: block;
width: 100%;
height: 50px;
border-radius: 25px;
outline: none;
border: none;
background-image: linear-gradient(to right, #32be8f, #38d39f, #32be8f);
background-size: 200%;
font-size: 1.2rem;
color: #fff;
font-family: 'Poppins', sans-serif;
text-transform: uppercase;
margin: 1rem 0;
cursor: pointer;
transition: .5s;
}
.btn:hover{
background-position: right;
}
@media screen and (max-width: 1050px){
.container{
grid-gap: 5rem;
}
}
@media screen and (max-width: 1000px){
form{
width: 290px;
}
.login-content h2{
font-size: 2.4rem;
margin: 8px 0;
}
.img img{
width: 400px;
}
}
@media screen and (max-width: 900px){
.container{
grid-template-columns: 1fr;
}
.img{
display: none;
}
.wave{
display: none;
}
.login-content{
justify-content: center;
}
}
Now, create a JavaScript file named main.js
and paste the specified codes into your JS file. Remember that you must create a file with the extension .js.
const inputs = document.querySelectorAll(".input");
function addcl(){
let parent = this.parentNode.parentNode;
parent.classList.add("focus");
}
function remcl(){
let parent = this.parentNode.parentNode;
if(this.value == ""){
parent.classList.remove("focus");
}
}
inputs.forEach(input => {
input.addEventListener("focus", addcl);
input.addEventListener("blur", remcl);
});
Conclusion
That’s all, now you’ve successfully created a animated and responsive Login Form with HTML, CSS and JavaScript. I hope it will be useful for you in future.
If you found this blog helpful, don’t forget to share it with others.